Hello
Latest Posts
C Language
Example 1
#include
int main()
{
/* Some list of combinations
0 = Black
1 = Blue
2 = Green
3 = Aqua
4 = Red
5 = Purple
6 = Yellow
7 = White
8 = Gray
9 = Light Blue
A = Light Green
B = Light Aqua
C = Light Red
D = Light Purple
E = Light Yellow
F = Bright White */
printf("This is a console color change program\n");
system("COLOR F2"); /* This will change the bgcolor F - White and textcolor to 2- Green */
getchar();
return 0;
}
Example 2
#include
#include
int main()
{
system("COLOR FC");
printf("Welcome to the color changing application!\n");
printf("Press any key to change the background color!\n");
getch();
system("COLOR 6C");
printf("Now the background color is Yellow and Text Color is light Red\n");
printf("Press any key to exit...");
getch();
return 0;
}
Example 3
#include //This is the header file for windows.
#include //C standard library header file
void SetColor(int ForgC){
WORD wColor;
//This handle is needed to get the current background attribute
HANDLE hStdOut = GetStdHandle(STD_OUTPUT_HANDLE);
CONSOLE_SCREEN_BUFFER_INFO csbi;
//csbi is used for wAttributes word
if(GetConsoleScreenBufferInfo(hStdOut, &csbi)){
//To mask out all but the background attribute, and to add the color
wColor = (csbi.wAttributes & 0xF0) + (ForgC & 0x0F);
SetConsoleTextAttribute(hStdOut, wColor);
}
}
int main()
{
printf("Test color"); //Here the text color is white
SetColor(4); //Function call to change the text color
printf("Test color"); //Now the text color is green
return 0;
}
agyanadda
January 30, 2019
In C-Language when you will use text color then you must have to know about the color code of C language.
Name | Value | Black | 0 Blue | 1 Green | 2 Cyan | 3 Red | 4 Magenta | 5 Brown | 6 Light Gray | 7 Dark Gray | 8 Light Blue | 9 Light Green | 10 Light Cyan | 11 Light Red | 12 Light Magenta| 13 Yellow | 14 White | 15 ----------------------- OR ----------------------- 0 = Black 1 = Blue 2 = Green 3 = Aqua 4 = Red 5 = Purple 6 = Yellow 7 = White 8 = Gray 9 = Light Blue A = Light Green B = Light Aqua C = Light Red D = Light Purple E = Light Yellow F = Bright White
Jquery
Bootstrap Example
agyanadda
January 27, 2019
You can create filter table in bootstrap using Jquery
Filter Tables
SR NO | EMPLOYEE NAME | ADDRESS | EMAIL ID |
---|---|---|---|
101 | Praveen | Noida | praveen@example.com |
102 | Nandan | Delhi | sam@mail.com |
103 | Deepti | Goa | deepti@greatstuff.com |
104 | Mohan | Delhi | mohan@test.com |
CPP
#include
using namespace std;
//create class
class Student{
public: // A.s
int id; //D.M
string nm; // D.M
//member function
void insert(int i, string n){
id=i;
nm=n;
}
void disp(){
cout<<"ID:"<
// Default Constructor Example
#include
using namespace std;
//create class
class Student{
public: // A.s
int id; //D.M
string nm; // D.M
//member function
void insert(){
cout<<"Enter ID & Name: ";
cin>>id>>nm;
}
void disp(){
cout<<"ID:"<
// Parametrized Constructor Example
#include
using namespace std;
//create class
class Student{
public:
int id;
string nm;
Student(int i, string n){
id=i;
nm=n;
}
void disp(){
cout<<"ID"<
agyanadda
January 24, 2019
PHP
Example 1
$color=array("red","Green","Blue");
//echo $color[1];
foreach($color as $val){
echo $val."
"; }
Example 2
$color=array("red","Green","Blue");
//echo $color[1];
foreach($color as $val){
echo $val."
"; }
Example 3
$array[0]="Shubham";
$array[1]="Rafiya";
foreach($array as $val){
echo $val."
"; } echo count($val);
Example 4
$col=array("abc","ccv","dsf");
echo count($col);
Example 5
$col=array("abc","ccv","dsf");
$arrlen=count($col);
for($i=0;$i<$arrlen;$i++){
echo $col[$i]."
"; }
Example 6
$col=array("abc","ccv","dsf");
$arrlen=count($col);
for($i=0;$i<$arrlen;$i++){
echo $col[$i]."
"; }
Example 1
$col['Green']="#123";
$col['Red']="#123";
$col['White']="#fff";
echo $col['Red'];
Example 2
$col=array("Green"=>"#123","White"=>"#231","Red"=>"#44");
echo $col['Green'];
Example 3
$col=array("Green"=>"#123","Green"=>"#231","Red"=>"#44");
echo $col['Green'];
Example 4
$col['Green']="#123";
$col['Red']="#123";
$col['White']="#fff";
foreach($col as $val=>$n){
echo $val."
"; }
Example 1
$arr=array(
array(1,2,3),
array(4,5,6),
array(7,8,9)
);
echo $arr[1][2];
}
Example 2
$arr=array(
array(1,2,3),
array(4,5,6),
array(7,8,9)
);
for($r=0;$r<3;$r++){
for($c=0;$c<3;$c++){
echo $arr[$r][$c];
}
echo "
"; } }
agyanadda
January 24, 2019
Array is used to stores multiple values in one single variable
We can create array by using "array" Keyword
Type of Array
- Indexed arrays - Numeric index
- Associative arrays -Named keys
- Multidimensional arrays - Contain one or more arrays
Indexed Array Examples
"; }
"; }
"; } echo count($val);
"; }
"; }
Associative Array Example
"; }
Multi Multidimensional Array Example
"; } }
C Language
/* TurboC Compiler */
#include
#include /*for clrscr()*/
int main(){
int num=100;
/*Use after declaration section*/
clrscr(); /*clear output screen*/
printf("value of num: %d\n",num);
return 0;
}
/* GCC compiler and TurboC Compiler For Windows */
#include
#include /*for system()*/
int main(){
int num=100;
/*Use after declaration section*/
printf("Not display due to clearscr");
system("cls"); /*clear output screen*/
printf("value of num: %d\n",num);
return 0;
}
/* gcc/g++ compiler Linux */
#include
#include /*for system()*/
int main(){
int num=100;
/*Use after declaration section*/
system("clear"); /*clear output screen*/
printf("value of num: %d\n",num);
return 0;
}
agyanadda
January 23, 2019
We can clear screen in C language according to the compiler.
- Using clrscr() - For TurboC Compiler
- Using system("cls") - For gcc/g++ & TurboC Compiler windows
- Using system("clear") - For gcc/g++ compiler in Linux
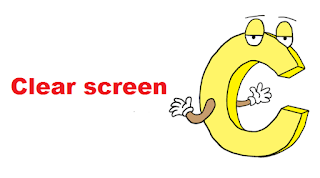
C Language
#include
#include
int main()
{
int arr[] = {1,2,3,4,5,6};
int length = sizeof(arr)/sizeof(int); //length of an integer array
printf("Array length = %d",length);
}
agyanadda
January 23, 2019
sizeof(): is used to get the data type size in C language.
Bootstrap, CSS
agyanadda
January 20, 2019
You can create bootstrap modal on page load only you have to write some script which is given below
Click Here To Live check
Auto Loading Bootstrap Modal on Page Load
Click Here To Live check
How to launch Bootstrap modal on page load
Bootstrap
agyanadda
January 20, 2019
How to create signup and login form in bootstrap
If you want to execute live then Click Login & signup form
Bootstrap Example
<If you want to execute live then Click Login & signup form
javascript
Validation Form using Javascript
agyanadda
December 29, 2018
How to validation using java script
Login Form Validation
Subscribe to:
Posts (Atom)